17 Mar 2015
With the cross section of the following technologies increasing amounts of code are being shared across multiple platforms.
- Portable Class Libraries
- Shared / Universal Apps & Projects
- Xamarin (Native)
- Xamarin.Forms
The idea that we can write our model (thinking in MVVM terms) once and share it across a wide array of platforms. There’s also some appeal to be able to share your view models in the same way.
With Caliburn.Micro we could do this already for the Microsoft XAML platforms. Your view models could be declared in a PCL that references Caliburn.Micro.Core and add something similar to the following code in your app.
ViewModelLocator.AddNamespaceMapping("Example.App.Views", "Example.App.Portable.ViewModels");
The use of Xamarin raises a lot questions.
- Can we share our view models on Xamarin platforms?
- What would Caliburn.Micro on Xamarin.Android and Xamarin.iOS even look like?
- How does Xamarin.Forms fit in to this?
Well the good news is that we’ve started work on bringing Caliburn.Micro to Xamarin and this post is to discuss a potential roadmap and pose some questions to you the community.
Below is a really simple breakdown of the feature-set of Caliburn.Micro and how it’s split between the Portable and Platform Assemblies.
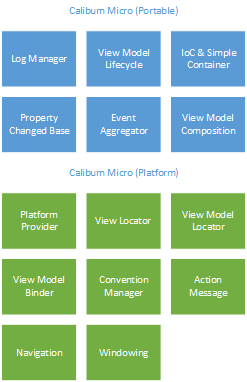
If we’ve built things right then everything that’s in the Portable assembly will already be ready for use in Xamarin apps. The only part that would be required in the Platform assembly is the piece highlighted below, the Platform Provider. This allows the Portable assembly to do things such as ensuring property changed notifications happen on the UI thread.
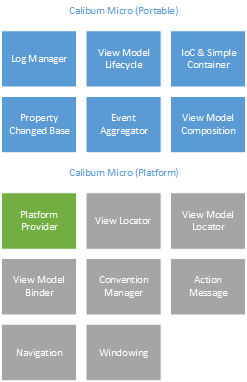
I’m really happy to say we have this mostly completed and available on the xamarin branch in GitHub. You can now share your view models across all the XAML platforms as well as Android and iOS!
You can see the start of some of these demonstrations at nigel-sampson/xamarin-experiements.
So where to from here? Part of the discussion I want to foster is which the grey boxes above make sense outside of a XAML framework and in what way do they make sense in these other platforms?
Could we add similar but not exactly the same features on these platforms such as ActivityLocator
instead or ViewLocator
?
Some of these features make a lot more sense when we consider Xamarin.Forms which is a XAML-like platform. We’d like to keep that as a separate assembly to ensure we don’t end up requiring certain editions of Xamarin. It would also need to be development in a way that Xamarin native development worked hand in hand with Xamarin.Forms.
We’re certainly not promising and exact version of Caliburn.Micro on Xamarin but the most we can speed up your development using similar patterns across multiple platforms the better we’ll all be.
The future is bright!
17 Mar 2015
We’ve just pushed 2.0.2 to nuget, it’s a small release mostly with bug fixes missing from 2.0.0.
What’s New?
- Resolved some design time bugs.
PropertyChangedBase.Refresh
is now virtual.
- Added checks to guard against an async CanClose.
- Allow
SettingsWindowManager
to open independent flyouts [WinRT].
- Deal with unicode in C# identifiers.
AttachedCollection.On*
methods are now virtual.
ViewAware.GetView
is now virtual`.
ViewLocator.LocateForModel
falls back to LocateForModelType
if the view could not be added to the visual tree.
ActionMessage.UpdateAvailablility
is now virtual.
- Removed explicit garbage collection from WinRT
FrameAdapter
.
- Resolved a bug where couldn’t navigate to a view model that contained the name of the assembly.
Thanks to all who contributed fixes, logged bugs etc.
14 Oct 2014
This is the start of something I’d like to do semi-regularly and highlight some of the community content out there about Caliburn.Micro.
Shows off some of the features and support for the Async / Await pattern brought in by C# 5.0 including the Event Aggregator and Coroutines
Demonstrates how you can use sample data at design time in both Expression Blend and Visual Studio and shows it working in a Universal app.
Discusses the process they went through migrating a 1.5 app to 2.0 in WPF.
A long series on using Caliburn.Micro in universal apps, in fact Matteo’s blog is fantastic resource for everything op the subject and recommend everyone have a browse.
MVVM Made Easy: an Intro to Caliburn Micro on Windows Phone
If you author and / or see any content out their that you think is worth highlighting then get in contact
20 Sep 2014
In my previous post I talked about how Caliburn.Micro deals with state on Windows Phone (Silverlight). Today I want to deal with the differences in WinRT (Windows 8 and Windows Phone 8.1) and how it impacts things. I want to finish with some potential solutions and open it up to discussion.
WinRT Frame View Caching
I’ve already talked about how the view caching behaviour of PhoneApplicationFrame
on Windows Phone (Silverlight) enables almost all the view state and view model state outcomes we’re expecting.
However the Frame
control in WinRT has a largely different behaviour which can trip a lot of developers (including myself) up.
The Page
control in WinRT has a property NavigationCacheMode
which by default is set to Disabled
. This means on every navigation a new view is created, including navigating back to a view you were previously on. This means we instantly lose any view and view model state upon navigation. You can see this happening in a brand new application where you scroll down a list, select and item to take you to another page. Upon navigating back you’ll notice the ScrollViewer
will be reset back to the start. Not desirable at all.
So what happens when we set NavigationCacheMode
to Enabled
?. Initially it looks like we get back to our desired behaviour, navigating back to our previous views restores a cached view rather than a new one. This view held our view model so view and view model state are retained.
The problems surface when you navigate forward again. For our first example lets consider an application with two views, Product List and Product Details. If the user taps a product and the Frame
navigates from Product List to Product Details. A new Product Details view and view model are created as expected, navigating back to Product List returns to the cached view / view model pairing with retained state.
Where it all goes wrong however is when the user taps a second product and the Frame
navigates to Product Details with a different parameter. The Frame
will reuse the cached view from the previous product. This means you’ll have the same view model from the previous product details as before as well. Unless you’re careful with your views the user will see a flash of the previous product details before the new product data loads.
This gets even worse if your application can potentially have the same view in the stack multiple times (for instance a file browser application may have a Browse Folder view). In this case with caching on then navigating backward or forward will be to the view / view model you were previously on and by loading the new state means you’re wiping out the state for the previous view so you get no benefit at all.
To summarise, the caching behaviour of the Frame
respects neither the type of navigation (Back, Forward, New) nor the parameter of the navigation. This is no where near our desired behaviour and puts a lot more onus on us as application developers to retain the correct state.
WinRT Frame State
Frame
does have one set of useful methods, GetNavigationState
and SetNavigationState
. GetNavigationState
returns a serialized representation of the forward and back stacks at the time of calling. It’s a custom format that you’re not supposed to understand, although some people have posted articles on manipulating it. One caveat is that this custom format can’t handle complex types when used as a navigation parameter and will throw an exception if they’re used. Simple types such as string and integer are fine
If you’re using INavigationService.UriFor<>
with simple types then you shouldn’t run into this problem.
We can use these methods to save frame state on suspension of the application, so if the application is terminated when can restore it to where the user was previously.
This functionality already exists in Caliburn.Micro and is usable through the methods INavigationService.SuspendState
and INavigationService.ResumeState
. These take the current frame state and store it in ApplicationData.Current.LocalSettings
to be restored if required.
You can see a sample of this in the Windows 8.1 Sample.
Potential Solutions
Selective Caching
For the Product List / Product Details example above we can get our desired behaviour by only enabling NavigationCacheMode
on the Product List view and not Product Details. This only works because we only have two levels of view, if for instance Product Details navigated forward to say Product Photo Gallery then this isn’t a plausible solution as we’ll want to enable caching on Product Details. We then run into the problems highlighted above.
For our File Browser example it makes sense to have NavigationCacheMode
set to Disabled
as we’re unable to retain any state at all given that it’s wiped out by the next view model on the stack. This doesn’t mean we get any of our desired behaviour, just lessen the amount of work we need to do to avoid a sub-optimal user experience.
Suspension Manager
The Visual Studio templates for Windows 8 and Windows Phone 8.1 come with a class SuspensionManager
than can help with some of these problems. First it gives us the ability to save the frame state of an application to allow for suspension and resumption of the application. It also maintains a “stack” of state for you. Because the default templates have a rather weird implementation of MVVM then you can use this state bucket for both view and view model state.
This avoids the requirement of enabling caching on the Frame, but pushes the responsibility back to you as a developer to determine which view / view model state your want to retain (including minor things like scroll position). This can get awkward when the ScrollViewer
is built into a GridView
and you can’t restore the scroll position until the layout is updated and so on. It’s a passable solution, but becomes onerous quickly and prone to mistakes.
Custom Frame
It’s occurred to me a number of times while ranting about this problem to create my own Frame control that acts in the same way as PhoneApplicationFrame
from Windows Phone (Silverlight). It’s certainly a possibility, but in my opinion on out of the scope of Caliburn.Micro in mandating control usage. Particular care would need to be paid around performance in caching views.
This is the best solution for retaining view state on Frame
navigation.
I still have hope some of this will be resolved in Windows 10.
Maintaining a View Model stack
An idea I’ve been experimenting with is having the Caliburn.Micro navigation service maintaining internal stacks of view models mapping one to one with the forward and back stacks of the Frame
. On navigating backwards a new view is created but an existing view model is reused. This would give us view model state retained over navigation. It’s working well in the experiments, but is running afoul of a weird xaml bug and cached views it would not help in the retaining of view state.
StorageHandler<> for WinRT
As quickly discussed in the previous post Caliburn.Micro has a solution to retain view model state on the current view model when the application is suspended through a class named StorageHander<>
(you can read more about it in the documentation). Porting this to WinRT is certainly a possibility, I have had some feedback the current API isn’t to everyone’s liking and it’s hard to gauge demand for this feature.
What this would do is allow you to record parts of your current view model’s state that would be stored somewhere in ApplicationData.Current.LocalSettings
for when the application is resumed. This would most likely be wired into the current methods on Navigation Service.
Get in touch
I’ve created an issue on GitHub to facilitate discussion around some of these issues and would invite feedback. Let us know what you think.
20 Aug 2014
In the previous post on application state we defined a whole of terms on how we can consider state and some of the user expectations how how our applications should respect that state. This this post I want to discuss what’s already in Caliburn.Micro for these problems in particular for the Windows Phone (Silverlight) platform.
Phone Application Frame
Applications in Windows Phone (Silverlight) are primarily view first, the root content of the app’s Window is a PhoneApplicationFrame
that Caliburn.Micro wraps in an INavigationService
. As the app navigates to new PhoneApplicationPage
’s our navigation service locates the appropriate view model for the view and activates it.
The PhoneApplicationFrame
maintains an internal cache of the views that lines up with the back and forward stacks. This means as you navigate back you’re returning the exact same view that you left earlier.
This behaviour gives us our desired outcomes for View State out of the box as that state is automatically preserved on the forward and back stacks. You can see this behaviour in existing apps by scrolling down a list, navigating forward and then back. All View State such as list scroll position should be maintained correctly.
The INavigationService
implementation FrameAdapter
takes care not to overwrite existing view models. Because views retain a reference to their view model (through DataContext) then these cached views act as a cache for the view model as well. Navigation back to a cached view causes an activation of the existing view model rather than creation.
In summary the view caching behaviour of PhoneApplicationFrame
coupled with Caliburn.Micro’s INavigationService
give us the desired behaviour right out of the box.
App Activation
App activation and deactivation has changed a bit over the course of Windows Phone versions with the introduction of fast app resume, but the basics remain the same.
Caliburn.Micro has some built in features to let you preserve some parts of a view model during the tombstoning of an application through the StorageHandler<T>
class. This implements the desired behaviour from part 1, you can read more about it in the documentation.
Where to from here?
In the next post I’ll discuss how we can go about addressing these same behaviours in the Windows RT platform.